RANDOM PAGE
SITE SEARCH
LOG
IN
SIGN UP
HELP
To gain access to revision questions, please sign up and log in.
A2
aAssembler 1
CTRL+Click here to run the simulator.
- Type in the code below or copy and paste it.
- Click the "A" button to assemble the code (translate it into binary).
- Click the "S" button to step through the program.
NOP
NOP
NOP
NOP
START:
NOP
NOP
NOP
NOP
JMP START
Step the program and watch the PC register carefully. Answer the reviseOmatic questions on this task.
- NOP is a command that does nothing for one processor clock cycle. It is used to provide tiny time delays.
- PC is the program counter.
- PC is a POINTER to the address of the instruction that is about to be executed.
- After each instruction, one is added to PC to make it point to the next instruction.
- There are a couple of exceptions to this rule.
- JMP (jump) commands make the program counter point to a completely new address.
- JMP START causes the program to jump to the Destination Address labelled by START:
TASK:
- Add a couple more labels and jumps.
- This is like the snakes and ladders game.
- You can jump forwards or backwards.
- Notice how all the jump addresses are automatically calculated for you when you assemble the code.
bAssembler 2
CTRL+Click here to run the simulator.
- Type in the code below or copy and paste it.
- Click the "A" button to assemble the code (translate it into binary).
- Click the "S" button to step through the program.
- Click the LEDs button on the highlighted "B" to connect the LEDs to PORTB.
- If necessary, drag the LEDs to a more visible position.
; CONNECT THE LEDs TO PORTB
MOVW 0x00
MOVWR TRISB
START:
MOVW 0x55
MOVWR PORTB
MOVW 0xAA
MOVWR PORTB
JMP START
|
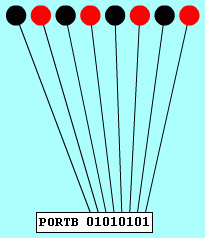
|
Step the program and watch the LEDs. Answer the reviseOmatic questions on this task.
- This program should also run unaltered on the PICAXE 28X1 chip but so fast that the LEDs will appear to be continuously lit. You will need to add time delays to see the LEDs flashing. Make sure you use resistors to limit the current to each LED.
- The microcontroller has three eight bit I/O ports called PORTA, PORTB and PORTC.
- The microcontroller has three data direction registers called TRISA, TRISB and TRISC.
- TRISA/B/C is a register controlling the tristate logic for PORTA/B/C.
- If TRISB bits are set to zero, the corresponding PORTB bits are outputs.
- If TRISB bits are set to one, the corresponding PORTB bits are inputs.
- MOVW is a command used to move a number into the Working Register (W)
- MOVWR TRISB is a command used to copy a number from (W) into the named register (TRISB)
- MOVWR 0x55 copies from (W) into the register with address 55.
- MOVWR PORTB is a command used to copy a number from (W) into the named register (PORTB)
- 0x55 is a hexadecimal number.
TASK: Alter the program to repeatedly flash each LED in turn going from right to left.
cAssembler 3
CTRL+Click here to run the simulator.
- Type in the code below or copy and paste it.
- Click the "A" button to assemble the code (translate it into binary).
- Click the "S" button to step through the program.
- Click the TRAFFIC button on the highlighted "C" to connect the traffic Lights to PORTC.
- If necessary, drag the lights to a more visible position.
; CONNECT TRAFFIC LIGHTS TO PORTC
MOVW 0x00
MOVWR TRISC
START:
MOVW 0x55 ; This number is wrong
MOVWR PORTC
MOVW 0xA ; This number is wrong
MOVWR PORTC
MOVW 0xC ; This number is wrong
MOVWR PORTC
MOVW 0x2 ; This number is wrong
MOVWR PORTC
JMP START
|
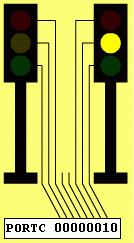
|
Step the program and watch the traffic lights. Answer the reviseOmatic questions on this task.
- This program should run unaltered on the PICAXE 28X1 chip.
- Time delays will need to be added or the lights will flash in a continuous blur.
- Remember to use LED current limiting resistors in the interface.
TASK: Work out the correct numbers needed to control the traffic lights. Use the grid below to design the correct number sequence.
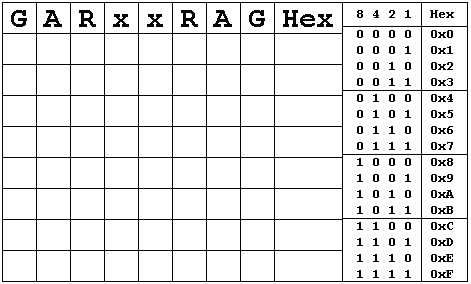
dAssembler 4
CTRL+Click here to run the simulator.
- Type in the code below or copy and paste it.
- Click the "A" button to assemble the code (translate it into binary).
- Click the "S" button to step through the program.
- Click on SEV-SEG and connect to PORTC.
- Step the program and watch the seven segment displays.
- Answer the reviseOmatic questions on this task.
; CONNECT THE DISPLAYS TO PORTC
MOVW 0X00 ; SET 8 PORTC OUTPUTS
MOVWR TRISC ; SET 8 PORTC OUTPUTS
MOVWR PORTC ; CLEAR RIGHT SEGMENTS
MOVW 0X80 ; CLEAR LEFT SEGMENTS
MOVWR PORTC ; CLEAR LEFT SEGMENTS
START:
MOVW 0X05
MOVWR PORTC
MOVW 0X85
MOVWR PORTC
MOVW 0X0
MOVWR PORTC
MOVW 0X80
MOVWR PORTC
JMP START
|
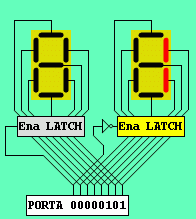
|
TASK: Easy: Make one of the digits count from 0 to 9.
TASK: Very Hard: Make both the digits count from 00 to 59 like the seconds and minutes on a clock.
Use this grid to help with the design of the binary control data.
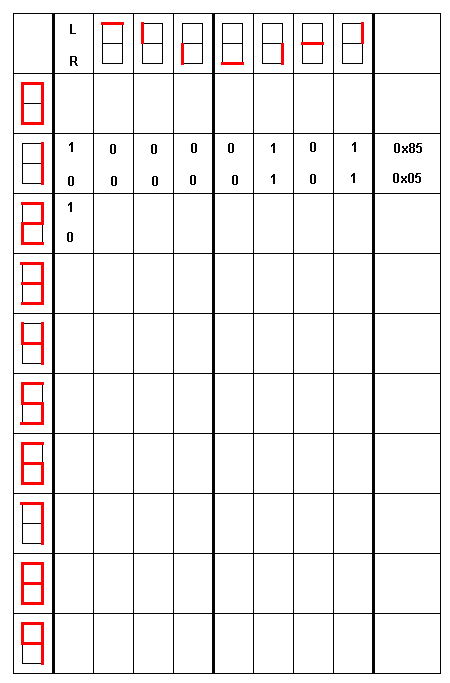
eAssembler 5
CTRL+Click here to run the simulator.
- Type in the code below or copy and paste it.
- Click the "A" button to assemble the code (translate it into binary).
- Click the "S" button to step through the program.
- Click the TRAFFIC button on the highlighted "C" to connect the traffic Lights to PORTC.
- If necessary, drag the lights to a more visible position.
- Don't cover SP or the stack, indicated by the blue highlight.
; =========================================
; TRAFFIC LIGHTS WITH TIME DELAY SUBROUTINE
; CONNECT THE LIGHTS TO PORTC
; =========================================
MOVW 0X00 ; 0: Output 1: Input
MOVWR TRISC ; PORTC 8 OUTPUTS
START:
MOVW 0X21 ; GARxxRAG - 00100001
MOVWR PORTC ; X X
MOVW 0X20 ; DELAY DURATION
CALL DELAY
MOVW 0X62 ; GARxxRAG - 01100010
MOVWR PORTC ; XX X
MOVW 0X05 ; DELAY DURATION
CALL DELAY
MOVW 0X84 ; GARxxRAG - 10000100
MOVWR PORTC ; X X
MOVW 0X20 ; DELAY DURATION
CALL DELAY
MOVW 0X46 ; GARxxRAG - 01000110
MOVWR PORTC ; X XX
MOVW 0X05 ; DELAY DURATION
CALL DELAY
JMP START
; =========================================
; ===== TIME DELAY SUBROUTINE =============
; =========================================
DELAY:
SUBW 0X1 ; SUBTRACT ONE FROM W
JPZ DONE ; JUMP IF Z FLAG IS SET
JMP DELAY ; CARRY ON COUNTING
DONE:
RET ; SUBROUTINE RETURN
; =========================================
|
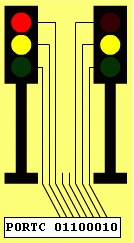
- CALL alters PC to the address labelled by DELAY:
- CALL also saves a "return address" on the Stack and SP is altered.
- SP is the stack pointer register. SP points to the next free stack location.
- SUBW 0x01 subtracts one from (W).
- JPZ jumps if the Z flag is set to 1.
- The Z flag is set if a move or a calculation leaves a Zero in W.
- JMP jumps whatever state the flags are in.
- RET sets PC to the address saved on the stack earlier.
|
This example uses a SUBROUTINE. Subroutines are small, self contained, re-usable modules within a program. They are easy to code and test because each subroutine performs a single, useful well defined task. In this program, it's a time delay.
When you CALL a subroutine, the processor needs to keep track of the address it has to return to when the subroutine is finished. The Stack is used to save these Return Addresses.
The stack is an area of memory used to store the return addresses of subroutine calls. The stack pointer is a register which points to the current stack position. Data is added and removed from the stack in a strict Last In First Out (LIFO) order. The stack pointer is adjusted to keep track of these operations.
Imagine a tall pile of dinner plates. It is easy to add to the top of the pile. Also it's easy to remove from the top of the pile. Removing a middle or bottom plate is not a good idea. The plate pile is a stack and obeys the LIFO rule.
When data is added to the stack this is called a PUSH. When data is removed from the stack it's called a POP. If you write bad code, the stack can grow so big that it "eats" your program. That would be a stack overflow. If you write your code correctly, each CALL has a matching RETurn. If you have more calls than returns, your stack will grow and eat your program. If you have more returns than calls, you will get a Stack Underflow. This might not destroy your program but something is going to break.
The Task ...
- Step the program and watch SP and the Stack.
- Answer the reviseOmatic questions on this task.
fAssembler 6
In this example switches are used to control the motor in an H Bridge configuration.
CTRL+Click here to run the simulator.
- Type in the code below or copy and paste it.
- Click the "A" button to assemble the code (translate it into binary).
- Click the "S" button to step through the program.
- Click on SWITCHES (B) and HBRIDGE (B) to make the switches and the motor visible.
- Both are connected to PORTB.
- If necessary, move the switches and motor so they don't cover each other.
; =======================================
; H Bridge Motor Control
; CONNECT HBRIDGE TO PORTB
; CONNECT SWITCHES TO PORTB
; =======================================
MOVW 0XF0 ; 4 Inputs & Outputs
MOVWR TRISB ; Set PORTB direction
START:
MOVRW PORTB ; READ PORTB
ANDW 0X70 ; BIT MASK
SUBW 0X10 ; TEST S4 CLOSED
JPZ FORWARD ; Jump If Closed
JMP COAST ; Freewheel
; =======================================
FORWARD:
MOVW 0X09 ; Close bits 0 and 3
MOVWR PORTB
JMP START
; =======================================
COAST:
MOVW 0X0 ; Open all switches
MOVWR PORTB
JMP START
; =======================================
|
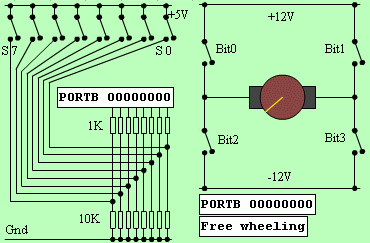
|
- Use your mouse to switch off all the switches 0 to 7. Just click on the switches.
- Turn on S4 only. The motor should run forwards.
- Turn off S4. The motor should free-wheel.
The Code
- MOVRW copies data from the specified location into the Working register. PORTB in this example.
- ANDW performs logical AND on the W register.
- Here it is used for a BIT MASK.
- Bits 0, 1, 2, 3 and 7 are forced to zero (masked) and bits 4, 5 and 6 are left unchanged.
- SUBW 0x10 subtracts hexadecimal 10 from W.
- If only switch 4 was closed, this operation will leave zero in W.
- This will cause the Z flag to be set.
- JPZ will perform the jump if the Z flag is set.
- The motor will run forwards.
TASK: Add to this example.
- If you close only switch 5, the motor should run in reverse.
- If you close only switch 6, the motor should brake to a halt.
- Switches 0, 1, 2, 3 and 7 should have no effect.
gAssembler 7
The TMR and PRE Registers
CTRL+Click here to run the simulator.
- Type in the code below or copy and paste it.
- Click the "A" button to assemble the code (translate it into binary).
- Click the "S" button to step through the program.
START:
; =========================================
; Initialise PRE and TMR
; =========================================
MOVW 0X1 ; Copy 1 into ...
MOVWR PRE ; ... the prescaler
MOVW 0X08 ; Copy 8 into ...
MOVWR TMR ; ... the timer
; =========================================
; Poll the TMR flag (T)
; =========================================
POLL:
MOVRW SR ; Copy SR into W
ANDW 0x02 ; Bit mask
JPZ POLL
; ==========================================
; == This code runs when TMR reaches zero ==
; ==========================================
NOP
NOP
NOP
NOP
JMP START
; ==========================================
- The AQA microcontroller contains a timer register called TMR.
- This simulator memory-maps this register at address 0xFF.
- There is also a prescaler register, PRE, memory-mapped at address 0xFE.
- NOTE: In AQA exams, these registers might not be memory mapped.
- If PRE contains zero, TMR does nothing.
- If PRE contains 10 (for example), on each clock pulse, a counter is incremented.
- When the counter reaches 10, one is subtracted from TMR and the counter reset to zero.
- This decrements TMR on every eleventh clock pulse.
- If PRE contains some larger number, the counter counts from zero to this larger number and then subtracts one from TMR.
- In this way, the clock frequency is divided by PRE + 1 and used to decrement TMR.
- When TMR reaches zero, the TMR (T) flag is set and stays set until a non zero value is assigned to TMR.
TASK: Copy and paste this code into the simulator and run the program.
- Note roughly how long it takes before the code controlled by the timer runs.
- Modify this code by storing 8 into PRE instead of 1.
- Run the modified code and note that the timer counts far slower and the timer events are less frequent.
hAssembler 8

CTRL+Click here to run the simulator.
- Type in the code below or copy and paste it.
- Click the "A" button to assemble the code (translate it into binary).
- Click the "S" button to step through the program.
- Click the "Gray" button and select Port C.
- Run the code with a clock frequency of 15Hz or more.
- Lower clock rates might allow the mechanism to overshoot before the code detects its position.
- Please answer the related reviseOmatic questions.
; ================================
; ===== GRAY CODE CONTROLLER =====
; ===== CONNECT TO PORTC =========
; ================================
MOVW 0X3F
MOVWR TRISC
FORWARD:
MOVW 0X40 ; Set bit 6
MOVWR PORTC
F_REP:
MOVRW PORTC
ANDW 0X3F
SUBW 0X0F
JPZ BACKWARD
JMP F_REP
BACKWARD:
MOVW 0X80 ; Set bit 7
MOVWR PORTC
B_REP:
MOVRW PORTC
ANDW 0X3F
SUBW 0X03
JPZ FORWARD
JMP B_REP
- 0x3F is copied to TRISC. This configures the PORTC inputs and outputs.
- 0x3F corresponds to OO I I I I I I where O is an output and I is an input.
- If bit 7 of PORTC is high, the motor drives the mechanism left.
- If bit 6 of PORTC is high, the motor drives the mechanism right.
- Bit 0 is the least significant bit of the Gray code pattern.
- Bit 5 is the most significant bit of the Gray code pattern.
- ANDW 0X3F This is a bit mask used to ignore bits 6 and 7
- SUBW 0X0F If W contains 0x3F, this subtraction leaves zero in W and the Z Flag is set.
- JPZ will jump, only if the Z Flag is set.
TASK:
- Alter the code so the mechanism goes approximately to the half way mark and back.
- Add code to make the mechanism reverse if it reaches the left or right hand ends of the scale.
iAssembler 9
CTRL+Click here to run the simulator.
- Type in the code below or copy and paste it.
- Click the "A" button to assemble the code (translate it into binary).
- Click the "S" button to step through the program.
- Click on STEPPER and select PORTB to make the motor visible.
- Please answer the reviseOmatic questions on this topic.
; ================================
; === CONNECT STEPPER TO PORTB ===
; ================================
start:
movw 0x1
movwr portb
nop
nop
movw 0x2
movwr portb
nop
nop
movw 0x4
movwr portb
nop
nop
movw 0x8
movwr portb
jmp start
|
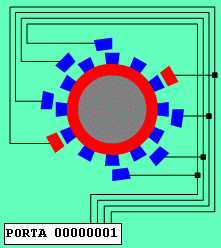
|
TASK:
- Alter the code so the motor runs backwards.
- Alter the code so the motor runs in half steps.
jAssembler 10
CTRL+Click here to run the simulator.
- Type in the code below or copy and paste it.
- Click the "A" button to assemble the code (translate it into binary).
- Click the "S" button to step through the program.
- Click on DAC and select PORTA to make the Summing DAC visible.
- D0 to D7 are DAC inputs. (Outputs on the microcontroller).
; ============================
; === CONNECT DAC TO PORTA ===
; ============================
movw 0x0
start:
movwr porta
addw 0x1
jmp start
TASK:
- What are the other resistor values?
- What is the resolution of this DAC?
- How many output values are possible?
|
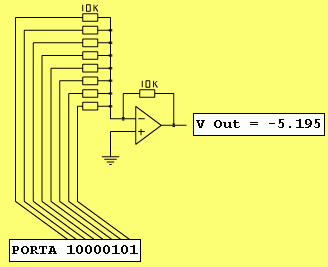
|
kAssembler 11
CTRL+Click here to run the simulator.
- Type in the code below or copy and paste it.
- Click the "A" button to assemble the code (translate it into binary).
- Click the "S" button to step through the program.
- Click on RAMP ADC and select PORTA to make the seven bit Digital Ramp ADC visible.
- Horizontal mouse movements alter the light level and the LDR output.
- D0 to D6 are ADC inputs. D7 is a ADC output, used to detect the end of conversion (EoC).
; =================================
; === CONNECT RAMP ADC TO PORTA ===
; =================================
jmp init
; ===== VARIABLES =====
count: db 0x00
delay: db 0x30
mask: db 0x80
; =====================
init:
movrw mask
movwr trisa
movw 0x0
movwr count
s:
movrw count
movwr porta
movrw porta
andw 0x80
jpz pause
inc count
jmp s
pause:
movrw delay
rep:
subw 0x1
jpz init
jmp rep
|
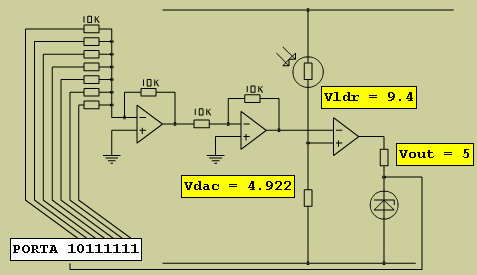
|
TASK:
- What are the other resistor values?
- What is the resolution of this DAC?
- How many output values are possible?
- Why is the inverting amplifier needed?
- What is the function of the comparator?
- Why is the Zener diode needed?
reviseOmatic V3
Contacts, ©, Cookies, Data Protection and Disclaimers
Hosted at linode.com, London